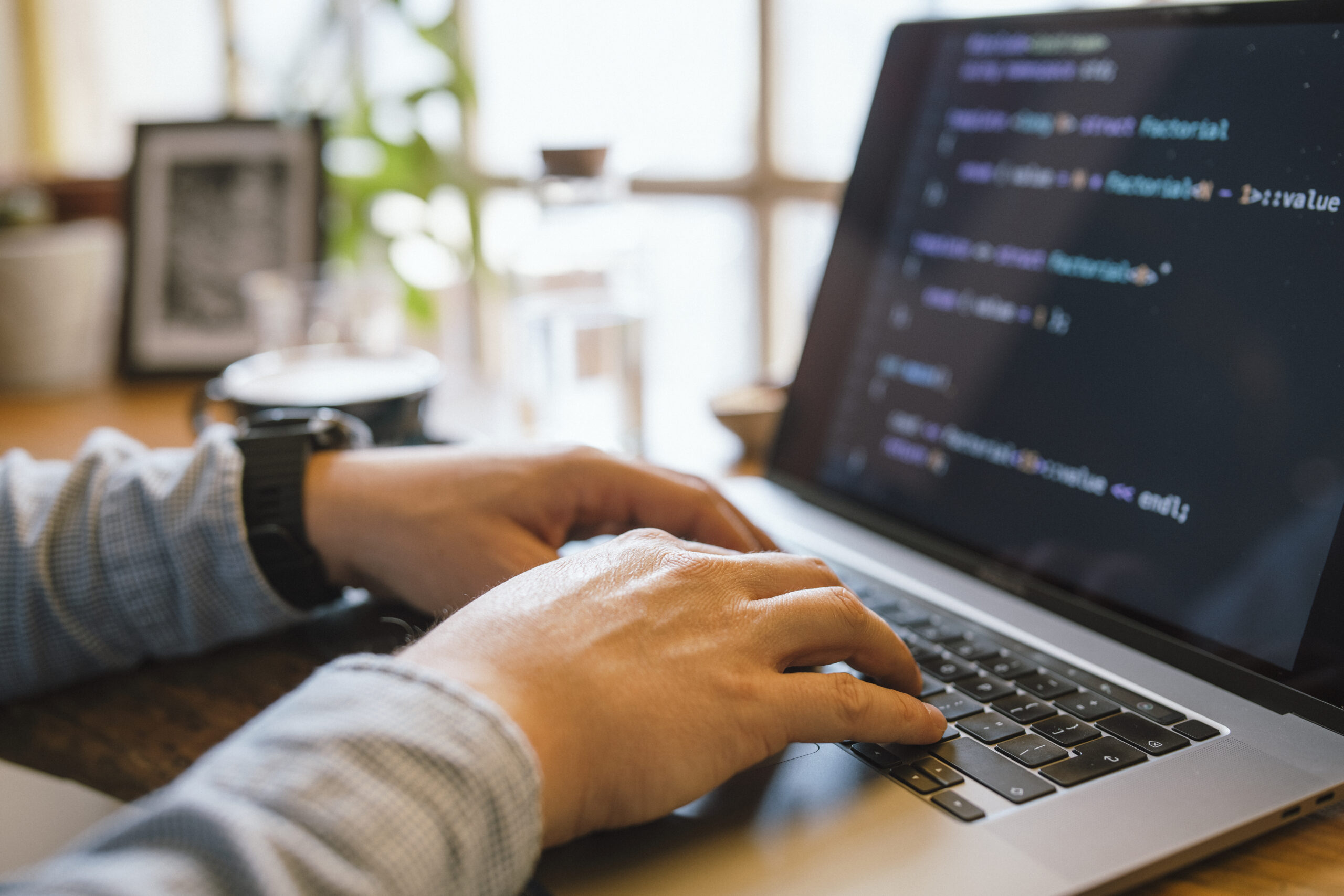
Debugging is one of the most vital — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or possibly a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies builders can elevate their debugging expertise is by mastering the instruments they use everyday. While crafting code is a person Component of growth, figuring out the way to communicate with it successfully during execution is Similarly significant. Modern-day enhancement environments occur Outfitted with effective debugging capabilities — but many builders only scratch the floor of what these equipment can perform.
Get, as an example, an Built-in Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, stage by means of code line by line, and in many cases modify code to the fly. When utilised appropriately, they Permit you to notice particularly how your code behaves for the duration of execution, that is a must have for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-end builders. They enable you to inspect the DOM, observe community requests, see true-time effectiveness metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can change frustrating UI troubles into manageable responsibilities.
For backend or technique-degree developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control in excess of functioning processes and memory administration. Studying these applications might have a steeper Finding out curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Command methods like Git to grasp code heritage, find the exact second bugs have been launched, and isolate problematic variations.
Ultimately, mastering your equipment indicates heading over and above default options and shortcuts — it’s about producing an personal expertise in your enhancement environment to ensure that when concerns come up, you’re not misplaced at midnight. The higher you understand your equipment, the more time you'll be able to devote fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently missed — techniques in productive debugging is reproducing the challenge. Just before jumping into the code or earning guesses, builders need to have to create a consistent environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What actions led to The problem? Which surroundings was it in — development, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise situations under which the bug happens.
Once you’ve gathered adequate information, try and recreate the issue in your neighborhood environment. This might mean inputting precisely the same data, simulating related user interactions, or mimicking program states. If The difficulty appears intermittently, look at writing automated checks that replicate the edge situations or point out transitions concerned. These assessments don't just aid expose the condition but additionally protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it might take place only on specified functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible circumstance, You should utilize your debugging applications extra correctly, test potential fixes safely, and communicate more clearly together with your team or customers. It turns an abstract criticism right into a concrete obstacle — Which’s the place developers prosper.
Browse and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As an alternative to viewing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the information thoroughly and in full. Quite a few developers, especially when underneath time strain, look at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it stage to a selected file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to recognize these can greatly quicken your debugging approach.
Some errors are vague or generic, As well as in those circumstances, it’s very important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
In the end, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint problems more rapidly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment within a developer’s debugging toolkit. When utilised proficiently, it offers true-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout improvement, INFO for typical gatherings (like prosperous start off-ups), WARN for potential challenges that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure critical messages and slow down your procedure. Target crucial events, point out adjustments, enter/output values, and significant choice points as part of your code.
Format your log messages Evidently and persistently. Consist of context, for instance timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re especially worthwhile in production environments the place stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging solution, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently discover and take care of bugs, builders should strategy the method just like a detective resolving a secret. This mindset assists break down sophisticated difficulties into workable sections and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Identical to a detective surveys against the law scene, collect just as much applicable information and facts as you can without jumping to conclusions. Use logs, exam conditions, and consumer studies to piece collectively a clear image of what’s happening.
Subsequent, form hypotheses. Ask yourself: What could possibly be leading to this behavior? Have any changes recently been made into the codebase? Has this difficulty happened ahead of beneath equivalent situations? The objective is to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled environment. When you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the final results direct you closer to the reality.
Spend shut consideration to little details. Bugs generally conceal during the minimum envisioned spots—like a missing semicolon, an off-by-one mistake, or a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can save time for potential challenges and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Writing exams is one of the best solutions to improve your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that provides you self confidence when generating improvements towards your codebase. A well-tested application is simpler to debug since it lets you pinpoint just the place and when a challenge happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Performing as predicted. Each time a examination fails, you right away know exactly where to appear, considerably reducing some time expended debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier becoming fixed.
Future, combine integration exams and end-to-conclusion assessments into your workflow. These assist ensure that several areas of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex devices with several factors or expert services interacting. If one thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is usually a powerful initial step. As soon as the test fails persistently, you can give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable method—serving to you capture more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the trouble—observing your monitor for several hours, trying solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Getting breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable glitches or misreading code you wrote just hrs previously. On this state, your brain becomes fewer economical at challenge-fixing. A short walk, a espresso split, and even switching to a special job for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken the perfect time to disconnect, allowing their subconscious perform within the background.
Breaks also enable avert burnout, Specifically during for a longer period debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Specifically less than tight deadlines, but it surely really brings about faster and simpler debugging Ultimately.
In brief, having breaks isn't a sign of weak spot—it’s a smart method. It presents your brain Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Understand From Each Bug
Each and every bug you click here face is a lot more than just a temporary setback—It really is a chance to mature as being a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and evaluate what went Mistaken.
Start out by inquiring you a few key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or comprehending and assist you to Develop stronger coding routines relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively stay clear of.
In staff environments, sharing That which you've realized from a bug with all your friends may be especially impressive. No matter if it’s by way of a Slack information, a brief create-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. After all, many of the very best builders aren't those who write best code, but those who repeatedly learn from their problems.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.